Our Approach to Counseling
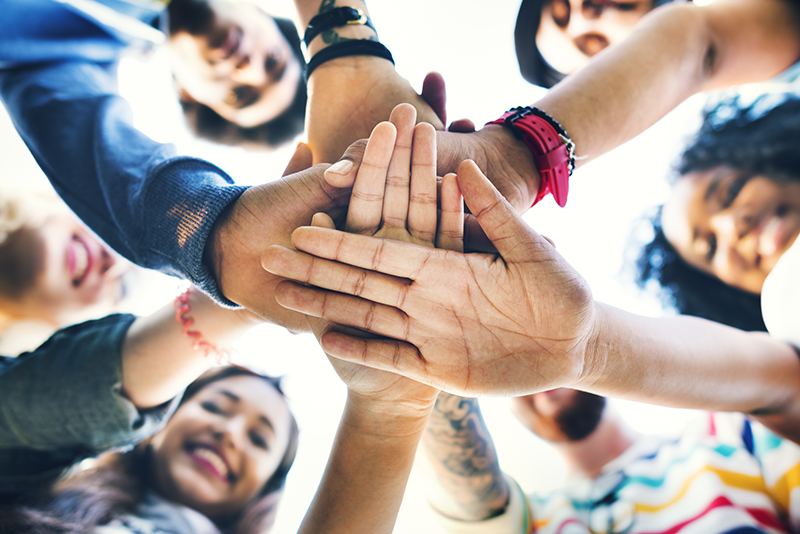
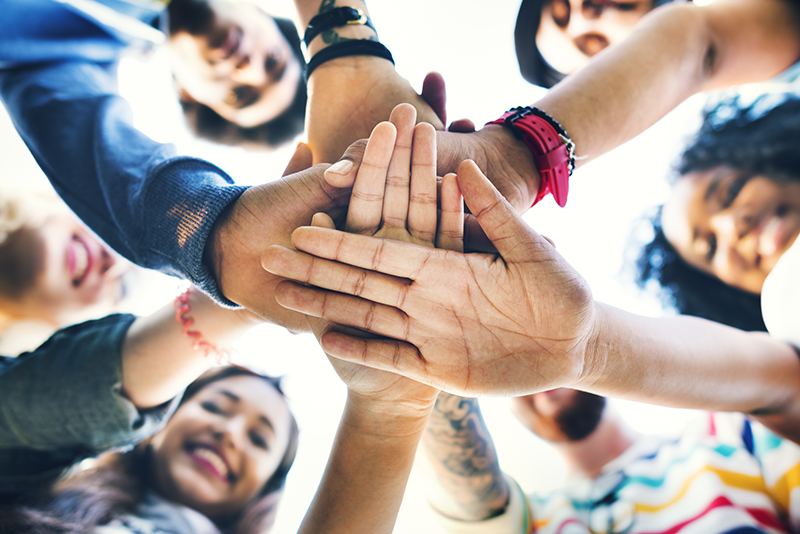
We believe you are a person -- not your mental illness. You are not your sadness, grief, anxiety or depression.
We'll explore these parts of your life, dig deeper and work together to find ways for you, the whole person, to achieve change.
Sometimes the process of exploring your difficulties can feel overwhelming. You're aware that something needs to change, but you're afraid of what might happen.
We know that the first step can be really hard, but the psychotherapist will serve as your guide. Yes, you will sometimes fall, or may be bumped and bruised along the way. But you can also hope to see great and wonderful things as we take this shared journey.
What Makes CPAS Unique:
The Benefits of Psychotherapy
Signs that you could benefit from therapy include:

Feeling Overwhelmed
You feel an overwhelming, prolonged sense of helplessness and sadness.

Excessive Worrying
You worry excessively, expect the worst or are constantly on edge.

Unresolved Problems
Your problems don't seem to get better despite your efforts and help from family and friends.